1. 개요
Spring Boot 애플리케이션에서 빈 의 하이브리드 정의는 어노테이션 기반 및 XML 기반 구성 을 모두 포함하는 것 입니다. 이 환경에서는 테스트 클래스에서 XML 기반 구성을 사용할 수 있습니다 . 그러나 때때로 이 상황에서 " Failed to load ApplicationContext "라는 애플리케이션 컨텍스트 로드 오류가 발생할 수 있습니다 . 이 오류는 애플리케이션 컨텍스트가 테스트 컨텍스트에 로드되지 않았기 때문에 테스트 클래스에 나타납니다.
이 예제에서는 XML 애플리케이션 컨텍스트를 Spring Boot 애플리케이션의 테스트에 통합하는 방법에 대해 설명합니다.
2. "ApplicationContext 로드 실패" 오류
Spring Boot 애플리케이션에 XML 기반 애플리케이션 컨텍스트를 통합하여 오류를 재현해 보겠습니다.
먼저 서비스 빈 정의 가 있는 application-context.xml 파일 이 있다고 가정해 보겠습니다 .
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="employeeServiceImpl" class="com.baeldung.xmlapplicationcontext.service.EmployeeServiceImpl" />
</beans>
이제 webapp/WEB-INF/ 위치 에 application-context.xml 파일을 추가할 수 있습니다 .
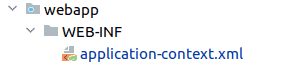
우리는 또한 서비스 인터페이스와 클래스를 만들 것입니다:
public interface EmployeeService {
Employee getEmployee();
}
public class EmployeeServiceImpl implements EmployeeService {
@Override
public Employee getEmployee() {
return new Employee("Baeldung", "Admin");
}
}
마지막으로 애플리케이션 컨텍스트에서 EmployeeService 빈 을 가져오기 위한 테스트 케이스를 생성합니다 .
@RunWith(SpringRunner.class)
@ContextConfiguration(locations={"classpath:WEB-INF/application-context.xml"})
public class EmployeeServiceAppContextIntegrationTest {
@Autowired
private EmployeeService service;
@Test
public void whenContextLoads_thenServiceISNotNull() {
assertThat(service).isNotNull();
}
}
이제 이 테스트를 실행하려고 하면 오류가 관찰됩니다.
java.lang.IllegalStateException: Failed to load ApplicationContext
이 오류는 애플리케이션 컨텍스트가 테스트 컨텍스트에 로드되지 않았기 때문에 테스트 클래스에 나타납니다. 또한 근본 원인은 WEB-INF 가 classpath에 포함되어 있지 않기 때문입니다 .
@ContextConfiguration(locations={"classpath:WEB-INF/application-context.xml"})
3. 테스트에서 XML 기반 ApplicationContext 사용하기
테스트 클래스에서 XML 기반 ApplicationContext 를 사용하는 방법을 살펴보겠습니다 . 테스트에서 XML 기반 ApplicationContext 를 사용하는 두 가지 옵션이 있습니다 : @SpringBootTest 및 @ContextConfiguration 어노테이션.
3.1. @SpringBootTest 및 @ImportResource 를 사용하여 테스트
Spring Boot는 @SpringBootTest 어노테이션을 제공 합니다. 이를 사용하여 테스트에서 사용할 애플리케이션 컨텍스트를 생성할 수 있습니다 . 또한 XML bean을 읽기 위해 Spring Boot 메인 클래스에서 @ImportResource 를 사용해야 합니다 . 이 어노테이션을 사용하면 빈 정의가 포함된 하나 이상의 리소스를 가져올 수 있습니다.
먼저 메인 클래스에서 @ImportResource 어노테이션을 사용합시다:
@SpringBootApplication
@ImportResource({"classpath*:application-context.xml"})
이제 애플리케이션 컨텍스트에서 EmployeeService 빈 을 가져오기 위한 테스트 케이스를 생성해 보겠습니다 .
@RunWith(SpringRunner.class)
@SpringBootTest(classes = XmlBeanApplication.class)
public class EmployeeServiceAppContextIntegrationTest {
@Autowired
private EmployeeService service;
@Test
public void whenContextLoads_thenServiceISNotNull() {
assertThat(service).isNotNull();
}
}
@ImportResource 어노테이션은 리소스 디렉터리 에 있는 XML 빈을 로드합니다. 또한 @SpringBootTest 어노테이션은 테스트 클래스에서 전체 애플리케이션의 빈을 로드합니다. 따라서 테스트 클래스에서 EmployeeService 빈에 액세스할 수 있습니다 .
3.2. 리소스 와 함께 @ContextConfiguration 을 사용 하여 테스트
테스트 구성 파일을 src/test/resources 디렉토리 에 배치 하여 다양한 구성의 빈으로 테스트 컨텍스트를 생성할 수 있습니다 .
이 경우 src/test/resources 디렉토리 에서 테스트 컨텍스트를 로드하기 위해 @ContextConfiguration 어노테이션을 사용합니다 .
먼저 EmployeeService 인터페이스에서 다른 빈을 생성해 보겠습니다.
public class EmployeeServiceTestImpl implements EmployeeService {
@Override
public Employee getEmployee() {
return new Employee("Baeldung-Test", "Admin");
}
}
그런 다음 src/test/resources 디렉토리 에 test-context.xml 파일을 생성합니다.
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="employeeServiceTestImpl" class="process.service.EmployeeServiceTestImpl" />
</beans>
마지막으로 테스트 케이스를 생성합니다.
@SpringBootTest
@ContextConfiguration(locations = "/test-context.xml")
public class EmployeeServiceTestContextIntegrationTest {
@Autowired
@Qualifier("employeeServiceTestImpl")
private EmployeeService serviceTest;
@Test
public void whenTestContextLoads_thenServiceTestISNotNull() {
assertThat(serviceTest).isNotNull();
}
}
여기 에서 @ContextConfiguration 어노테이션 을 사용하여 test-context.xml 에서 employeeServiceTestImpl 을 로드했습니다.
3.3. WEB-INF 와 함께 @ContextConfiguration 을 사용한 테스트
WEB-INF 디렉토리 에서 테스트 클래스의 애플리케이션 컨텍스트를 가져올 수도 있습니다 . 이를 위해 파일 URL을 사용하여 애플리케이션 컨텍스트를 처리할 수 있습니다.
@RunWith(SpringRunner.class)
@ContextConfiguration(locations = "file:src/main/webapp/WEB-INF/application-context.xml")
4. 결론
이 기사에서는 Spring Boot 애플리케이션의 테스트 클래스에서 XML 기반 구성 파일을 사용하는 방법을 배웠습니다. 항상 그렇듯이 이 기사에 사용된 소스 코드는 GitHub에서 사용할 수 있습니다 .